It’s more fun to create simple
application in VB.Net with less amount of code. Just simply drag and drop controls.
Since, Visual Basic.Net provides features where you can create quick
application in a minute. In this tutorial, I’ll show you how to create simple
calculator in Visual Basic.Net. Unfortunately, it only covers very simple
calculation with four arithmetic operators such as Addition, Subtraction,
Multiplication, and Division.
Here is the sample screenshot.
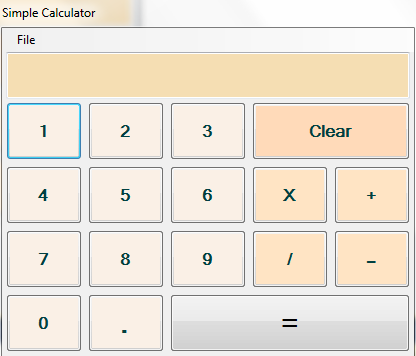 |
Simple Calculator in Visual Basic.Net |
Source code:
Option Explicit On
Public Class frmcalculator
Dim number1 As Single
Dim number2 As Single
Dim answer As Single
Dim arithmeticoperator As
String
Private Sub
btn1_Click(sender As System.Object, e As
System.EventArgs) Handles
btn1.Click
If txtdisplaynumber.Text = "0"
Then
txtdisplaynumber.Text = "1"
Else
txtdisplaynumber.Text = txtdisplaynumber.Text & "1"
End If
End Sub
Private Sub
btn2_Click(sender As System.Object, e As
System.EventArgs) Handles
btn2.Click
If txtdisplaynumber.Text = "0"
Then
txtdisplaynumber.Text = "2"
Else
txtdisplaynumber.Text = txtdisplaynumber.Text & "2"
End If
End Sub
Private Sub
btn3_Click(sender As System.Object, e As
System.EventArgs) Handles
btn3.Click
If txtdisplaynumber.Text = "0"
Then
txtdisplaynumber.Text = "3"
Else
txtdisplaynumber.Text = txtdisplaynumber.Text & "3"
End If
End Sub
Private Sub
btn4_Click(sender As System.Object, e As
System.EventArgs) Handles
btn4.Click
If txtdisplaynumber.Text = "0"
Then
txtdisplaynumber.Text = "4"
Else
txtdisplaynumber.Text = txtdisplaynumber.Text & "4"
End If
End Sub
Private Sub
btn5_Click(sender As System.Object, e As
System.EventArgs) Handles
btn5.Click
If txtdisplaynumber.Text = "0"
Then
txtdisplaynumber.Text = "5"
Else
txtdisplaynumber.Text = txtdisplaynumber.Text & "5"
End If
End Sub
Private Sub
btn6_Click(sender As System.Object, e As
System.EventArgs) Handles
btn6.Click
If txtdisplaynumber.Text = "0"
Then
txtdisplaynumber.Text = "6"
Else
txtdisplaynumber.Text = txtdisplaynumber.Text & "6"
End If
End Sub
Private Sub
btn7_Click(sender As System.Object, e As
System.EventArgs) Handles
btn7.Click
If txtdisplaynumber.Text = "0"
Then
txtdisplaynumber.Text = "7"
Else
txtdisplaynumber.Text = txtdisplaynumber.Text & "7"
End If
End Sub
Private Sub
btn8_Click(sender As System.Object, e As
System.EventArgs) Handles
btn8.Click
If txtdisplaynumber.Text = "0"
Then
txtdisplaynumber.Text = "8"
Else
txtdisplaynumber.Text = txtdisplaynumber.Text & "8"
End If
End Sub
Private Sub
btn9_Click(sender As System.Object, e As
System.EventArgs) Handles
btn9.Click
If txtdisplaynumber.Text = "0"
Then
txtdisplaynumber.Text = "9"
Else
txtdisplaynumber.Text = txtdisplaynumber.Text & "9"
End If
End Sub
Private Sub
btn0_Click(sender As System.Object, e As
System.EventArgs) Handles
btn0.Click
If txtdisplaynumber.Text = "0"
Then
txtdisplaynumber.Text = "0"
Else
txtdisplaynumber.Text = txtdisplaynumber.Text & "0"
End If
End Sub
Private Sub
Button14_Click(sender As System.Object, e As
System.EventArgs) Handles
Button14.Click
txtdisplaynumber.Text = txtdisplaynumber.Text & "."
End Sub
Private Sub
btnplus_Click(sender As System.Object, e As
System.EventArgs) Handles
btnplus.Click
number1 = Val(txtdisplaynumber.Text)
txtdisplaynumber.Text = "0"
arithmeticoperator = "+"
End Sub
Private Sub
btnx_Click(sender As System.Object, e As
System.EventArgs) Handles
btnx.Click
number1 = Val(txtdisplaynumber.Text)
txtdisplaynumber.Text = "0"
arithmeticoperator = "x"
End Sub
Private Sub
btndivide_Click(sender As System.Object, e As
System.EventArgs) Handles
btndivide.Click
number1 = Val(txtdisplaynumber.Text)
txtdisplaynumber.Text = "0"
arithmeticoperator = "/"
End Sub
Private Sub
btnminus_Click(sender As System.Object, e As
System.EventArgs) Handles
btnminus.Click
number1 = Val(txtdisplaynumber.Text)
txtdisplaynumber.Text = "0"
arithmeticoperator = "-"
End Sub
Private Sub
btnequals_Click(sender As System.Object, e As
System.EventArgs) Handles
btnequals.Click
number2 = Val(txtdisplaynumber.Text)
'Addition
If arithmeticoperator = "+"
Then
answer = number1 + number2
End If
'Subtraction
If arithmeticoperator = "-"
Then
answer = number1 - number2
End If
'Multiplication
If arithmeticoperator = "x"
Then
answer = number1 * number2
End If
'Division
If arithmeticoperator = "/"
Then
answer = number1 / number2
End If
'Result
txtdisplaynumber.Text = answer
End Sub
Private Sub btnclear_Click(sender
As System.Object,
e As System.EventArgs)
Handles btnclear.Click
txtdisplaynumber.Text = "0"
'Enable to clear
End Sub
Private Sub
ExitToolStripMenuItem_Click(sender As System.Object, e As
System.EventArgs) Handles
ExitToolStripMenuItem.Click
Me.Close()
End Sub
End Class